If you have a reasonable understanding of how to build modern websites using HTML, CSS & JavaScript then the Electron framework makes it extremely easy to build desktop applications for both Windows and macOS. As an introduction to the framework we’ll be creating a simple desktop clock application.
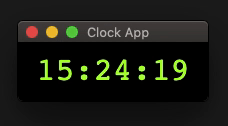
Before getting started it’s recommended to have a current version of node.js installed.
Ok, first thing we’ll do is create the folder/file structure required for our application:
electron-test/
├─ package.json
├─ index.js
├─ index.html
├─ script.js
├─ style.css
package.json
This file Indicates which command to run when we start
the application:
{
"name": "electron-test",
"main": "index.js",
"scripts": {
"start": "electron ."
}
}
Code language: JSON / JSON with Comments (json)
Note: Don’t use "name": "electron"
or the Electron installation will fail.
Install Electron
Open up a new terminal window in the project folder and then run the install:
npm install --save-dev electron
This downloads all the required node modules and adds the dev dependency to our package.json
.
index.js
This file is used to create windows and handle system events.
For our clock app we’ll create a small (190×80) fixed size browser window:
const { app, BrowserWindow } = require("electron");
app.whenReady().then(createWindow);
function createWindow() {
const win = new BrowserWindow({
width: 190,
height: 80,
resizable: false,
});
win.loadFile("index.html");
}
Code language: JavaScript (javascript)
index.html
Basic HTML file that loads the CSS and JS for the the clock functionality:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Clock App</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<script src="script.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
script.js
Fetch’s the current time and updates it each second (1000 milliseconds) in the index.html
.
function getTime() {
time = new Date().toLocaleTimeString();
document.body.innerHTML = time;
}
setInterval(getTime, 1000);
Code language: JavaScript (javascript)
style.css
Lastly the CSS to improve the appearance of our clock:
body {
font-family: monospace;
font-size: 32px;
background-color: black;
color: greenyellow;
text-align: center;
}
Code language: CSS (css)
Using a monospace
font here prevents the clocks position shifting as the numbers change.
Start the application
We can now start our application by running the following command:
npm start
Hopefully you’ve enjoyed this introduction to Electron and it inspires you to start building your own desktop applications.