In this tutorial we’ll be using JavaScript to generate a deck of playing cards and then selecting a random card from that deck. We’ll then output the random card into the browser and add some CSS so it looks like an actual playing card. If you’re interested in building card games using JavaScript this is a good starting point before moving onto building more complex card games.
Let’s get started by creating a index.html
file with the following markup:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Random Playing Card</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<script src="script.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
Then create a script.js
file and add a deckBuilder
function. This function will be used to generate an array with the values of 52 playing cards:
function deckBuilder() {
// ...
}
Code language: JavaScript (javascript)
Inside the deckBuilder
function we’ll create 2 arrays. First an array with the number/letter values found on standard playing cards, followed by an array with the 4 suits:
const values = [ "A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", ];
const suits = ["Hearts", "Diamonds", "Spades", "Clubs"];
Code language: JavaScript (javascript)
Next still inside the deckBuilder
function we’ll loop through the suits
array whilst also looping through the values
array to create and object with a card value and suit. We then push the combined data into the cards
array:
const cards = [];
for (let s = 0; s < suits.length; s++) {
for (let v = 0; v < values.length; v++) {
const value = values[v];
const suit = suits[s];
cards.push({ value, suit });
}
}
return cards;
Code language: JavaScript (javascript)
We now have a function that will create an array with the suit/value combination for all 52 playing cards. If you run console.log(deckBuilder());
you should see data for all of the cards structured like the following:
0: {value: "A", suit: "Hearts"}
1: {value: "2", suit: "Hearts"}
2: {value: "3", suit: "Hearts"}
Code language: CSS (css)
Next we’ll create a randomCard()
function and pass it the cards
array:
function randomCard(cards) {
// ...
}
const cards = deckBuilder();
randomCard(cards);
Code language: JavaScript (javascript)
Inside the randomCard
function we’ll generate a random number between 0 and 51 (not 52 as array indexes start at 0) and then get the data from the cards
array at that index. This data is then stored in variables to make it easier to work with later:
const random = Math.floor(Math.random() * 51);
const cardValue = cards[random].value;
const cardSuit = cards[random].suit;
Code language: JavaScript (javascript)
As there are HTML character entities for each of the playing card suits (♥/♦/♠/♣)
we can output these onto our playing card without having to use images. Because the entity code for diamonds is truncated we need to also truncate the "Diamonds"
string. The other suits entity codes match the strings (♥ ♠ ♣) so all we need todo is convert them to lowercase.
Add the following to the randomCard
function:
let entity;
cardSuit === "Diamonds" ? (entity = "♦") : (entity = "&" + cardSuit.toLowerCase() + ";");
Code language: JavaScript (javascript)
Finally complete the randomCard
function by rendering the random card into the HTML page:
const card = document.createElement("div");
card.classList.add("card", cardSuit.toLowerCase());
card.innerHTML =
'<span class="card-value-suit top">' + cardValue + entity + '</span>' +
'<span class="card-suit">' + entity + '</span>' +
'<span class="card-value-suit bot">' + cardValue + entity + '</span>';
document.body.appendChild(card);
Code language: JavaScript (javascript)
That’s all for the JavaScript functionality, we can now add some CSS so the HTML outputted looks like an actual playing card. Create a file called style.css
and add the following to first create the basic playing card shape:
.card {
position: relative;
width: 105px;
height: 150px;
border-radius: 5px;
border: 1px solid #ccc;
box-shadow: 0px 0px 5px 0px rgba(0,0,0,0.25);
}
Code language: CSS (css)
Because .card
is positioned relatively we can use absolute positioning for the top and bottom text. We’ll also rotate the text at the bottom 180 degrees just like a real playing card.
.card-value-suit {
display: inline-block;
position: absolute;
}
.card-value-suit.top {
top: 5px;
left: 5px;
}
.card-value-suit.bot {
transform: rotate(180deg);
bottom: 5px;
right: 5px;
}
.card-suit {
font-size: 50px;
position: absolute;
top: 50%;
transform: translateY(-50%);
left: 25%;
}
Code language: CSS (css)
And finally we’ll add the colors for each of the suits:
.card.spades,
.card.clubs {
color: black;
}
.card.hearts,
.card.diamonds {
color: red;
}
Code language: CSS (css)
Open the index.html
file in a browser and you should see a random playing card. Each time the page is refreshed a new random playing card will be generated that should look something like the following:
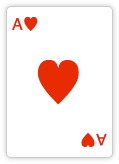
There you have it, you’ve just learnt how to build a webpage that loads a random playing card using JavaScript. As always the source code for this project can be found on GitHub. If you enjoyed this tutorial why not check out more of our practical web development tutorials in the links below.