In this tutorial you’ll learn how to build a realtime JavaScript chat application that uses a Firebase to store the messages. We’ll be taking advantage of the Firebase “realtime” database which allows us to synchronise realtime data without having to write any server code.
Setup the project in Firebase
If you haven’t already you’ll need to create a free Firebase account . Once logged into your account goto the console and add a new project called “Realtime Chat”. Disable the Google Analytics as this isn’t required.
Once the setup is complete you’ll be taken to a screen which has an option to add Firebase to your app, select the “Web” option and follow the prompts.
To complete the setup we need to add a database which is done by selecting “Realtime Database” from the sidebar menu. When prompted for the security rules select “Start in test mode”.
Coding the realtime chat app
We can now move onto coding the app starting with the HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Chatterbox</title>
</head>
<body>
<div id="chat">
<ul id="messages"></ul>
<form id="send-message">
<input id="chat-txt" type="text" />
<button id="chat-btn" type="submit">Submit</button>
</form>
</div>
<script src="https://www.gstatic.com/firebasejs/8.2.1/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.2.1/firebase-database.js"></script>
<script src="script.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
Next create a new script.js
file and add the Firebase config for your project. This code can be found in the “Project Settings” accessible from the main menu in Firebase, it’ll look something like the following:
const firebaseConfig = {
apiKey: "AIzaSyCSVtkMotInxYNE-fnvfVdaC5aVGlhzj4k",
authDomain: "realtime-chat-57589.firebaseapp.com",
projectId: "realtime-chat-57589",
storageBucket: "realtime-chat-57589.appspot.com",
messagingSenderId: "937988465303",
appId: "1:937988465507:web:ccf97g5919226364f8a956",
};
firebase.initializeApp(firebaseConfig);
const db = firebase.database();
Code language: JavaScript (javascript)
We then need a way for users to enter their username. To keep things simple we’ll use a prompt
to capture the data:
const username = prompt("What's your name?");
Code language: JavaScript (javascript)
Next we’ll use an event listener to call a postChat
function on form submission. This function saves the data to the messages collection in Firebase using a timestamp to seperate each record:
document.getElementById("send-message").addEventListener("submit", postChat);
function postChat(e) {
e.preventDefault();
const timestamp = Date.now();
const chatTxt = document.getElementById("chat-txt");
const message = chatTxt.value;
chatTxt.value = "";
db.ref("messages/" + timestamp).set({
usr: username,
msg: message,
});
}
Code language: JavaScript (javascript)
Here’s what the data looks like once saved to the database:
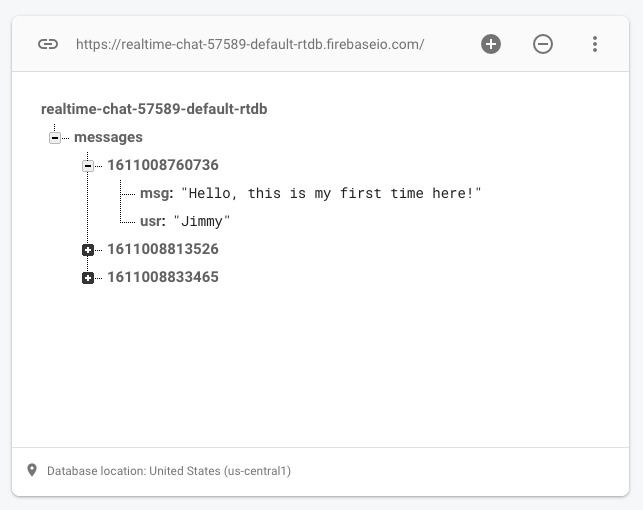
With the chat messages now being stored in the database we just need to render the messages into the HTML. Firebase has a built in child_added
event that is triggered once for each existing message and then again every time a new message is added:
const fetchChat = db.ref("messages/");
fetchChat.on("child_added", function (snapshot) {
const messages = snapshot.val();
const msg = "<li>" + messages.usr + " : " + messages.msg + "</li>";
document.getElementById("messages").innerHTML += msg;
});
Code language: JavaScript (javascript)
Now whenever a message is submitted a new list item with the username and message is appended to the end of the messages list. If you open the application in seperate browser tabs you’ll see that messages update in realtime.
Hopefully you’ve enjoyed building this simple JavaScript realtime chat app. Firebase really does make it easy to build applications that require realtime functionality. I’d also recommend checking out the Firebase Community to learn more about the full range of features available in Firebase.