In this tutorial you’ll learn how to develop a coming soon landing page with a JavaScript countdown timer. This page could be used to display the time remaining until a big event, website launch, or product release.
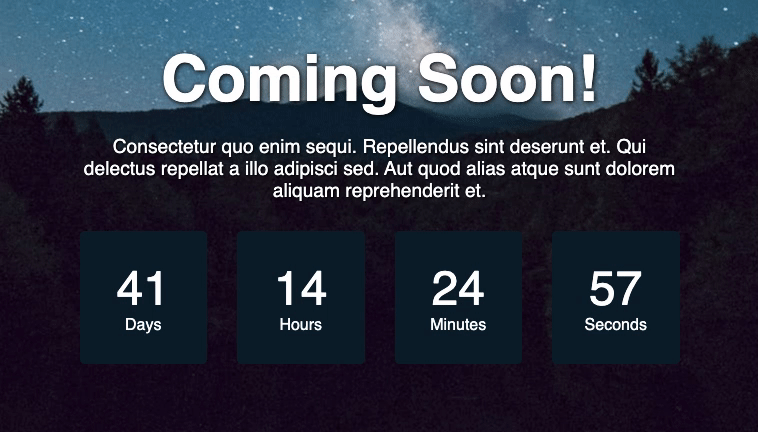
Let’s get started by creating a new HTML file with the following markup:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Hello World</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div id="countdown">
<h1>Coming Soon!</h1>
<p>Consectetur quo enim sequi. Repellendus sint deserunt et. Qui delectus repellat a illo adipisci sed. Aut quod alias atque sunt dolorem aliquam reprehenderit et.</p>
<ul>
<li><span id="days"></span>Days</li>
<li><span id="hours"></span>Hours</li>
<li><span id="minutes"></span>Minutes</li>
<li><span id="seconds"></span>Seconds</li>
</ul>
</div>
<script src="script.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
Next create a script.js
file and start by defining variables for each of the units of time:
(() => {
const sec = 1000,
min = sec * 60,
hour = min * 60,
day = hour * 24;
})();
Code language: JavaScript (javascript)
As we’re building a countdown timer we’ll need to specify an end date/time using the following format:
const end = new Date("Jul 01, 2021 12:00:00").getTime();
Code language: JavaScript (javascript)
To calculate the time remaining we’ll use setInterval
to fetch the current time every 1000 milliseconds. We can then calculate the time remaining by subtracting it from the end date and update the HTML text:
const int = setInterval(() => {
const current = new Date().getTime();
const remaining = end - current;
document.getElementById("days").innerText = Math.floor(remaining / day);
document.getElementById("hours").innerText = Math.floor( (remaining % day) / hour );
document.getElementById("minutes").innerText = Math.floor( (remaining % hour) / min );
document.getElementById("seconds").innerText = Math.floor( (remaining % min) / sec );
}, 1000);
Code language: JavaScript (javascript)
Currently when the countdown expires the timer will continue to run showing the time passed since the end date. Instead we’ll set all the digits to 0 and update the text to indicate the end date has been reached. To do this add the following to the end of the setInterval
method:
if (remaining < 0) {
document.querySelector("h1").innerText = "We Have Arrived!";
document.querySelector("p").innerHTML = "The big day is finally here - view our <a href=https://www.website.com>website<a/> for more information.";
const digit = document.querySelectorAll("span");
digit.forEach((digit) => {
digit.innerText = "0";
});
clearInterval(int);
}
Code language: JavaScript (javascript)
That completes the countdown functionally now for the CSS.
So the countdown is centrally aligned both horizontally and vertically the body has been set to display: flex
with the alignment centered. A background image was sourced from Pexels and set to display full screen using the CSS cover
property:
html {
height: 100%;
overflow: hidden;
}
body {
background: url("https://images.pexels.com/photos/1252869/pexels-photo-1252869.jpeg?auto=compress&cs=tinysrgb&dpr=2&h=650&w=940") no-repeat center center fixed;
color: #fff;
font-family: sans-serif;
text-align: center;
min-height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
Code language: CSS (css)
For the typography we’ll add a text-shadow
as it makes it easier to read when overlaid on a background image, we’ll also increase the font sizes and override the default blue link color:
h1 {
font-size: 4rem;
text-shadow: 1px 1px 5px #000;
margin: 0;
}
p {
font-size: 1.2rem;
text-shadow: 1px 1px 2px #000;
}
a {
color: #fff;
}
Code language: CSS (css)
Lastly the numbers and text in the countdown timer. As there are four elements we’ll use flex: 25%
to distribute them evenly on the horizontal axis. So the numbers stand out we’ll add a background color (taken from the background image):
#countdown {
max-width: 600px;
}
#countdown ul {
margin: 5% 0 0 0;
padding: 0;
display: flex;
gap: 5%;
}
#countdown ul li {
flex: 25%;
padding: 5%;
margin: 0;
list-style: none;
background: #001f2f;
border-radius: 5px;
}
#countdown ul li span {
display: block;
font-size: 3rem;
}
Code language: CSS (css)