In the past client side HTML form validation could only be achieved by using JavaScript. While HTML form validation isn’t as customisable as a JavaScript solution it’s a much simpler to implement.
Let’s go over some of the different way to validate forms with HTML.
Required Fields
If a field is mandatory you just need to add the required
attribute:
<input id="name" type="text" required>
Code language: HTML, XML (xml)
Email validation
Email addresses can be validated using a type="email"
input field. This checks that the inputed text contains both @ and . symbols with no spaces between the characters.
<input id="email" type="email" name="email" required>
Code language: HTML, XML (xml)
Username validation
Username requirements vary so we need to use the pattern
attribute. This attribute allows us to use regular expressions to test for more explicit requirements.
In this example the pattern only allows letters, numbers, and be between 3 & 15 characters in length:
<input id="username" type="text" name="username" required pattern="^[a-z0-9]{3,15}$" title="Password may only contain letters and numbers">
Code language: HTML, XML (xml)
Also note the inclusion of the title
attribute. This will appear in a tooltip to help users understand the field requirements if the text entered isn’t valid.
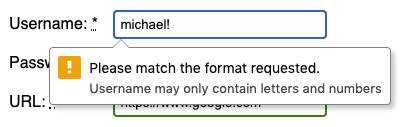
Password validation
Just like with usernames the requirements for passwords vary so we’ll once again use a pattern to define the validation rules. This pattern will accept between 8 & 25 characters and include a uppercase letter, lowercase letter, and a number:
<input id="password" type="password" name="password" required pattern="^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)[a-zA-Z\d]{8,25}$">
Code language: HTML, XML (xml)
URL validation
URLs can be validated using the type="url"
input field. An optional pattern is used to ensure URLs start with http(s) otherwise file:// would be accepted a valid URL:
<input id="url" type="url" name="url" required pattern="https?://.+">
Code language: HTML, XML (xml)
Age validation
If you need to validate an age or any number that falls between two values the type="number"
input is used. As number fields only allow numeric characters we just need to specify a min and max values:
<input id="age" type="number" name="age" required min="18" max="36">
Code language: HTML, XML (xml)
Styling valid/invalid input using CSS
Using the following CSS we can style fields based on whether they contain valid or invalid data:
input {
border: 2px solid #eee;
border-radius: 4px;
}
input:valid,
input:in-range {
border-color: green;
}
input:invalid,
input:out-of-range {
border-color: red;
}
Code language: CSS (css)
If a field contains a valid input it will have a “green” border otherwise the border is “red”.
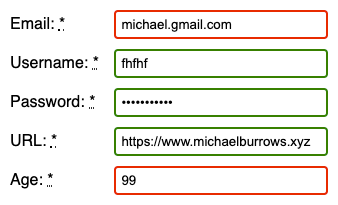
That’s all for this tutorial. We covered some of the most common validation requirements but as you’ve seen the pattern attribute combined with REGEX allows for very specific validation requirements.