In this tutorial we’ll be building a React component that fetches the current Bitcoin price from an API. The data source used is https://blockchain.info/ticker which is free with no account or access token required.

We’ll be working with the following files in the /src
folder:
├ BitcoinPrice.js
├ BitcoinPrice.css
├ bitcoin-logo.png
Code language: plaintext (plaintext)
You can download a free Bitcoin logo to use from icons8.com.
Let’s start by setting up the imports and function in the BitcoinPrice.js
file:
import React, { useState, useEffect } from "react";
import logo from "./bitcoin-logo.png";
import "./BitcoinPrice.css";
const BitcoinPrice = () => {
//...
};
export default BitcoinPrice;
Code language: JavaScript (javascript)
Next in the BitcoinPrice
function define the price
and loading
state:
const BitcoinPrice = () => {
const [price, setPrice] = useState(null);
const [loading, setLoading] = useState(true);
//...
};
Code language: JavaScript (javascript)
We’ll make the API request using a vanilla JavaScript fetch()
method:
const BitcoinPrice = () => {
const [price, setPrice] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch("https://blockchain.info/ticker")
.then((res) => res.json())
.then((data) => {
console.log(data);
setPrice(data.USD.last);
setLoading(false);
})
.catch((error) => {
console.log(error);
});
}, []);
//...
};
Code language: JavaScript (javascript)
Complete the function by adding the return statement containing the data and HTML:
const BitcoinPrice = () => {
const [price, setPrice] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch("https://blockchain.info/ticker")
.then((res) => res.json())
.then((data) => {
setPrice(data.USD.last);
setLoading(false);
})
.catch((error) => {
console.log(error);
});
}, []);
return (
<div className="btc">
<img className="btc-logo" src={logo} alt="Bitcoin" />
<span className="btc-price">
{loading ? "LOADING" : "$" + price}
</span>
</div>
);
};
Code language: JavaScript (javascript)
Finally add the following CSS to the BitcoinPrice.css
file:
.btc {
background-color: darkcyan;
display: flex;
justify-content: center;
align-items: center;
height: 60px;
}
.btc-logo {
height: 25px;
margin-right: 5px;
}
.btc-price {
color: #fff;
font-size: 18px;
font-weight: bold;
font-family: sans-serif;
}
Code language: CSS (css)
That’s all for this tutorial. You should now have a functioning component that fetches the current bitcoin price in $USD. The API also provides the price in many other currencies if you interested in building an application for international users.
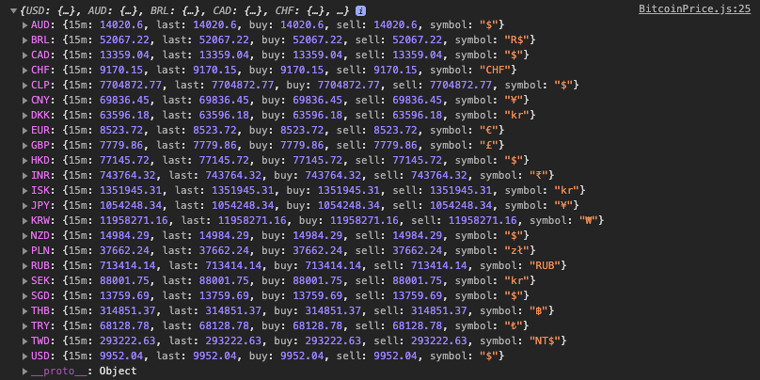