SortableJS is a JavaScript library for building reorder-able drag and drop UI components. As an introduction to the library we’ll build a simple quiz component that will require the user to correctly order a set of answers using drag and drop.
Here’s how the completed component will look and function in the browser:
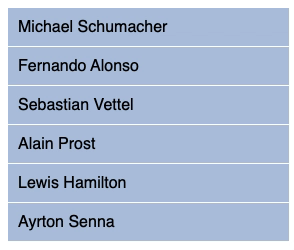
Let’s get started by creating a new HTML file and load in the SortableJS library via CDN:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>Drag & drop quiz component with SortableJS</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/sortablejs@latest/Sortable.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
In our quiz we’ll be asking the user to sort a list of F1 drivers by their number of Grand Prix victories. For this we’ll need to create an unordered list with the driver names. We’ll also add a button that the user can click once they are happy with the order selected to check it’s correct:
<h1>Sort the drivers by their number of Grand Prix victories:</h1>
<ul id="quiz">
<li>Ayrton Senna</li>
<li>Sebastian Vettel</li>
<li>Lewis Hamilton</li>
<li>Fernando Alonso</li>
<li>Michael Schumacher</li>
<li>Alain Prost</li>
</ul>
<button onclick="checkAnswer()">Check Answer</button>
Code language: HTML, XML (xml)
Next create a script.js
file and initiate SortableJS by passing the id (quiz) of the list:
new Sortable(quiz);
Code language: JavaScript (javascript)
The correct order of the answers is stored in array as follows:
const correctAnswers = [
"Michael Schumacher",
"Lewis Hamilton",
"Sebastian Vettel",
"Alain Prost",
"Ayrton Senna",
"Fernando Alonso",
];
Code language: JavaScript (javascript)
We also need a function for when the “Check Answer” button is clicked:
function checkAnswer() {
const li = document.querySelectorAll("#quiz li");
let answers = new Array();
li.forEach(function (text) {
answers.push(text.innerHTML);
});
if (JSON.stringify(correctAnswers) === JSON.stringify(answers)) {
alert("Correct :)");
} else {
alert("Try Again...");
}
}
Code language: JavaScript (javascript)
This function creates an answers
array from each of the list items in the quiz and then compares it with the correctAnswers
array. If the two arrays match we display as simple “Correct” alert message otherwise the user is greeted with a “Try Again” message. You can test the functionality yourself now in a browser.
Finally let’s create a file called style.css
and add the following CSS:
body {
font-family: sans-serif;
}
#quiz {
width: 360px;
margin: 0 0 15px 0;
padding: 0;
}
#quiz li {
display: block;
background-color: lightsteelblue;
padding: 10px;
border-bottom: 1px solid #fff;
cursor: move;
}
#quiz li.sortable-chosen {
background-color: lightslategray;
}
Code language: CSS (css)
Using cursor:move;
changes the cursor type when a list item that is draggable is hovered over, this helps to let the user know that these elements can be dragged. Applying a background color when a list item is chosen is also important here as it makes it easier to know where the selected list item will drop once the mouse is released.
In this tutorial we’ve built a simple drag and drop quiz component, however SortableJS has many more features that allow you to create even more elaborate drag and drop functionality. To explore these features check out the official documentation that can be found here.