ES6 provides the ability to split JavaScript into multiple files (modules). These modules can then be imported & exported as required into other files. This allows developers to organise project with many different JavaScript files.
To better understand how modules work let’s create a simple project that uses imports and exports. To get started create the following files and folder structure:
|- index.html
|- app.js
|- /src
|- location.js
|- weather.js
Then add the following markup to the index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Our Application</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="app.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
Notice the type="module"
so that the browser treats the script as a module, it wont work without it.
Exporting
Before we can import we must first export the code from the weather.js
and location.js
files. In weather.js
let’s create a weather()
function with an export
directive:
export function weather() {
const temperature = "15c";
const conditions = "Sunny"
return temperature + " " + conditions;
}
Code language: JavaScript (javascript)
And in location.js
we’ll export multiple variables by adding the desired members to the export
directive:
const country = "Australia";
const state = "Victoria";
const city = "Melbourne";
export { country, state, city };
Code language: JavaScript (javascript)
Importing
Now in the app.js
file we can import the external JavaScript.
import { country, state, city } from "./src/location.js";
import { weather } from "./src/weather.js";
Code language: JavaScript (javascript)
Once imported the code can be used just as it would if it existed in a single JavaScript file. To complete the project we’ll output the imported modules data into the index.html
.
const getWeather = weather();
const currentWeather = "<h2>Weather " + getWeather + "</h2>";
const currentLocation = "<h1>" + country + " | " + state + " | " + city + "</h1>";
document.getElementById("app").innerHTML = currentLocation + currentWeather;
Code language: JavaScript (javascript)
You should see the following when index.html
is viewed in a browser.
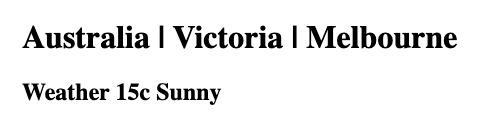
Note: Modules will only work in files served via http(s) not in the local file system (file:///).