In this tutorial you’ll learn how to fetch data from a remote API and output that data into a HTML page. Learning to work with API data is a crucial skill to learn as a web developer. Many websites and applications rely on internal or external APIs to provide data that’s displayed in the frontend.
The API we’ll be using is TheCocktailDB a free API with over 600 cocktail recipes and can be used without the need to create an account. If cocktails aren’t your thing there’s also TheSportsDB, TheAudioDB, and TheMealDB, all of which are also free and publicly available.
We’ll be building a page that fetches a random cocktail recipe like the following:
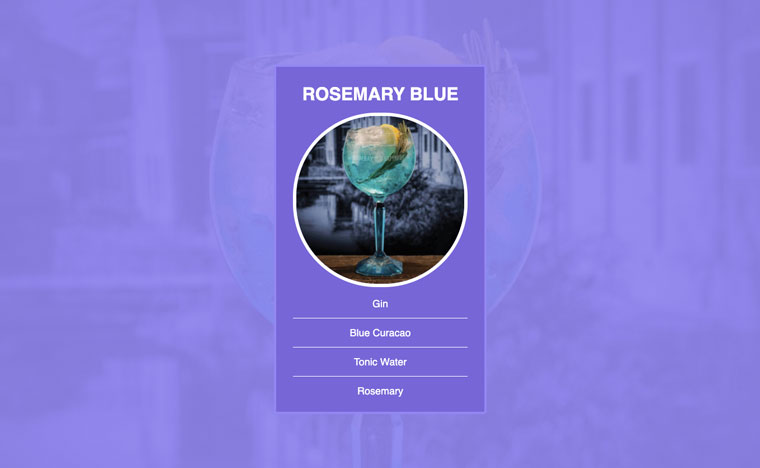
Let’s get started by creating a HTML file with the following markup:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Random Cocktail Recipe</title>
<link href="style.css" rel="stylesheet" />
</head>
<body>
<div id="cocktail"></div>
<div id="overlay"></div>
<script src="script.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
Along with loading the CSS and JavaScript we’ve included 2 empty divs. The first one will be used to inject the cocktail data from the API whilst the second will be used to achieve the semi transparent background effect that can be seen above.
Next in the script.js
file we’ll make our request using the Fetch API
. Currently supported in all browsers excluding IE and Opera Mini. The Fetch API provides a simple interface for fetching HTTP resources:
fetch("https://www.thecocktaildb.com/api/json/v1/1/random.php")
.then((response) => {
if (response.ok) {
return response.json();
} else {
throw new Error("NETWORK RESPONSE ERROR");
}
})
.then(data => {
console.log(data);
displayCocktail(data)
})
.catch((error) => console.error("FETCH ERROR:", error));
Code language: JavaScript (javascript)
This script performs the fetch request to the CocktailDB API endpoint. If the request was successful the result is stored as a JSON object, otherwise an error response message is returned. We then log the data to the console to make testing and debugging easier and call a displayCocktail
function that will include the functionality to output the data as HTML.
If the request was successful you’ll be able to view the data in the console:
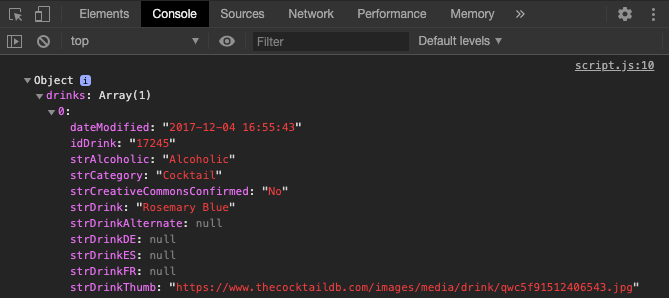
We can now pass this data to the function that will render it into the HTML. Create a displayCocktail()
function in script.js
and declare some variables for the data
and the <div>
that’ll be used to output the data:
function displayCocktail(data) {
const cocktail = data.drinks[0];
const cocktailDiv = document.getElementById("cocktail");
}
Code language: JavaScript (javascript)
Now let’s output the data into our HTML starting with a heading containing the cocktail name:
function displayCocktail(data) {
const cocktail = data.drinks[0];
const cocktailDiv = document.getElementById("cocktail");
// cocktail name
const cocktailName = cocktail.strDrink;
const heading = document.createElement("h1");
heading.innerHTML = cocktailName;
cocktailDiv.appendChild(heading);
}
Code language: JavaScript (javascript)
Next let’s get the image and append it to the cocktail <div>
. We’ll also use this image as the background for the <body>
function displayCocktail(data) {
const cocktail = data.drinks[0];
const cocktailDiv = document.getElementById("cocktail");
// cocktail name
const cocktailName = cocktail.strDrink;
const heading = document.createElement("h1");
heading.innerHTML = cocktailName;
cocktailDiv.appendChild(heading);
// cocktail image
const cocktailImg = document.createElement("img");
cocktailImg.src = cocktail.strDrinkThumb;
cocktailDiv.appendChild(cocktailImg);
document.body.style.backgroundImage = "url('" + cocktail.strDrinkThumb + "')";
}
Code language: JavaScript (javascript)
The ingredients are more difficult to output as they’re not stored in an array we can easily loop through. To get around this we’ll create an object and only add the ingredients that don’t have a null
value:
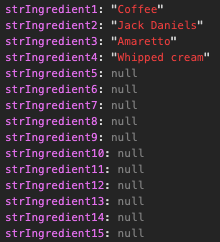
We can then loop through these ingredients and output them into an unordered list:
function displayCocktail(data) {
const cocktail = data.drinks[0];
const cocktailDiv = document.getElementById("cocktail");
// cocktail name
const cocktailName = cocktail.strDrink;
const heading = document.createElement("h1");
heading.innerHTML = cocktailName;
cocktailDiv.appendChild(heading);
// cocktail image
const cocktailImg = document.createElement("img");
cocktailImg.src = cocktail.strDrinkThumb;
cocktailDiv.appendChild(cocktailImg);
document.body.style.backgroundImage = "url('" + cocktail.strDrinkThumb + "')";
// cocktail ingredients
const cocktailIngredients = document.createElement("ul");
cocktailDiv.appendChild(cocktailIngredients);
const getIngredients = Object.keys(cocktail)
.filter(function (ingredient) {
return ingredient.indexOf("strIngredient") == 0;
})
.reduce(function (ingredients, ingredient) {
if (cocktail[ingredient] != null) {
ingredients[ingredient] = cocktail[ingredient];
}
return ingredients;
}, {});
for (let key in getIngredients) {
let value = getIngredients[key];
listItem = document.createElement("li");
listItem.innerHTML = value;
cocktailIngredients.appendChild(listItem);
}
}
Code language: JavaScript (javascript)
That concludes the required JavaScript for this tutorial.
Now all that’s left to do is add some CSS to the style.css
file:
html {
height: 100%;
}
body {
display: flex;
justify-content: center;
align-items: center;
height: 100%;
background-size: cover;
font-family: sans-serif;
}
#overlay {
background: rgba(147, 135, 242, 0.9);
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
z-index: -1;
}
#cocktail {
max-width: 350px;
text-align: center;
padding: 30px 30px 12px 30px;
color: #fff;
background-color: #7766d7;
border: 4px solid #9387f2;
border-radius: 5px;
}
#cocktail h1 {
margin: 0 0 15px 0;
text-transform: uppercase;
}
#cocktail img {
max-width: 300px;
border: 6px solid #fff;
border-radius: 150px;
}
#cocktail ul {
list-style: none;
margin: 0;
padding: 0;
}
#cocktail li {
padding: 15px 0;
font-size: 18px;
}
#cocktail li:not(:last-of-type) {
border-bottom: 1px solid #fff;
}
Code language: CSS (css)
That’s all for this this tutorial, you should have a functioning webpage that displays a random cocktail recipe from an external API. If you would like to learn more about the Fetch API and all the options available the MDN web docs provide an excellent resource.