Google Sheets can be used to provide a makeshift database that’s easy to modify for non-developers. It’s not the best solution for high traffic sites but works well for internal websites or when prototyping an app.
In this tutorial we’ll use Papa Parse to fetch data from a Google Sheet into React.
Let’s start by installing Papa Parse into our React application using NPM:
npm install papaparse
For this tutorial I’ve created a simple spreadsheet with the following data:
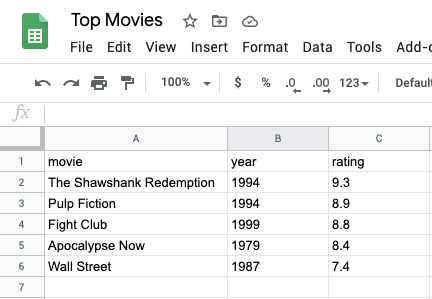
There are some requirements for the structure of this data:
- All columns must have a “label” in the first row and not contain any weird characters.
- Google assumes an empty row is the end of the sheet and stops returning data.
Once the sheet is complete select “File” -> “Publish to web” so it’s publicly visible.
Now for the component, create a new MovieData.js file with the following imports:
import React, { useState } from "react";
import Papa from "papaparse";
Code language: JavaScript (javascript)
Next create a MovieData()
function and declare a data
variable that will store the data:
const MovieData = () => {
const [data, setData] = useState({});
}
export default MovieData;
Code language: JavaScript (javascript)
We can now fetch the data from the Google Sheet, we just need to supply the spreadsheet URL to Papa Parse. If successful the results are saved to the data
State. We then convert this data into an array so we can parse it into our HTML:
const MovieData = () => {
const [data, setData] = useState({});
Papa.parse("https://docs.google.com/spreadsheets/d/1SJ8LxWmaxKBTgDJLvfD9NZLctBT931x19--qH2yLxck/pub?output=csv", {
download: true,
header: true,
complete: (results) => {
setData(results.data);
},
});
const movies = Array.from(data);
return (
// TODO: Output data as HTML
);
};
export default MovieData;
Code language: JavaScript (javascript)
Replace the URL with your Spreadsheet URL ensuring pub?output=csv
remains.
Finally lets return the movie data by adding the following to the return statement:
...
return (
<ul>
{movies.map((data) => (
<li key={data.movie}>
{data.movie} ({data.year}) - Rating {data.rating}
</li>
))}
</ul>
);
...
Code language: HTML, XML (xml)
You’ll notice movie
, year
, and rating
all correspond with text in the columns of the first row of the spreadsheet. If you’re using this code with a different spreadsheet you’ll simply need to modify this as required inline with the different data.
That’s all for this tutorial. You should now be able to fetch data in a React application from Google Sheets. As always you can download the full working source code for this tutorial from GitHub.