In this tutorial you’ll learn how to schedule cron jobs in Node.js. Typically cron jobs are used to automate system maintenance but can also be used for scheduling file downloads or sending emails at regular intervals.
Let’s first setup our project by running the following commands :
mkdir cron-jobs
cd cron jobs
npm init -y
We’ll be using the node-cron package which simplifies creating cron jobs in node.js using the full crontab syntax. Run the following command to install node-cron
:
npm install node-cron
With node-cron
installed create a new index.js
file with a sample cron job that will run every minute:
var cron = require("node-cron");
cron.schedule("* * * * *", () => {
console.log("Running each minute");
});
Code language: JavaScript (javascript)
The asterisks are part of the crontab syntax used to represent different units of time. Five asterisks represents the crontab default which will run every minute.
Here’s what unit of time each of the asterisks represent and the values allowed:
┌──────────────── second (optional 0 - 59)
| ┌────────────── minute (0 - 59)
| | ┌──────────── hour (0 - 23)
| | | ┌────────── day of month (1 - 31)
| | | | ┌──────── month (1 - 12)
| | | | | ┌────── day of week (0 - 7, 0 or 7 are sunday)
| | | | | |
| | | | | |
* * * * * *
Code language: plaintext (plaintext)
Schedule cron jobs daily/weekly/monthly
Run at midnight every day:
cron.schedule("0 0 * * *", () => {
// task to run daily
});
Code language: JavaScript (javascript)
Run every Sunday at midnight:
cron.schedule("0 0 * * 0", () => {
// task to run weekly
});
Code language: JavaScript (javascript)
Run on the first day of every month at midnight:
cron.schedule("0 0 1 * *", () => {
// task to run monthly
});
Code language: JavaScript (javascript)
If you’re struggling to understand exactly how the crontab syntax works check out crontab guru. This website provides a simple editor that displays the cron schedule based on the cron syntax you input:
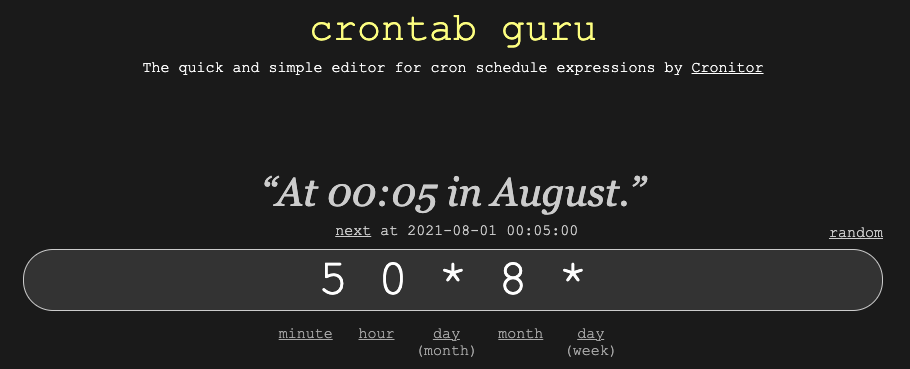
That’s all for this tutorial. Hopefully you’ll now be able to a setup your own cron job to save time on things you may have done manually in the past. As always thanks for reading!